Find out how HackerEarth can boost your tech recruiting
Learn more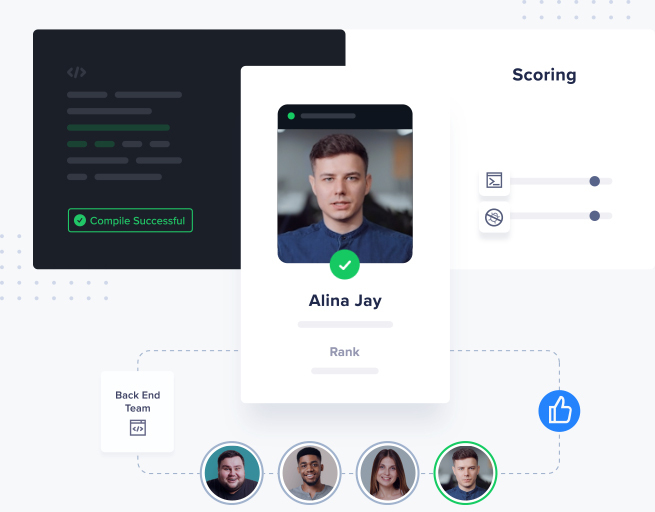
6 Best Practices to Design Javascript Coding Challenges with APIs
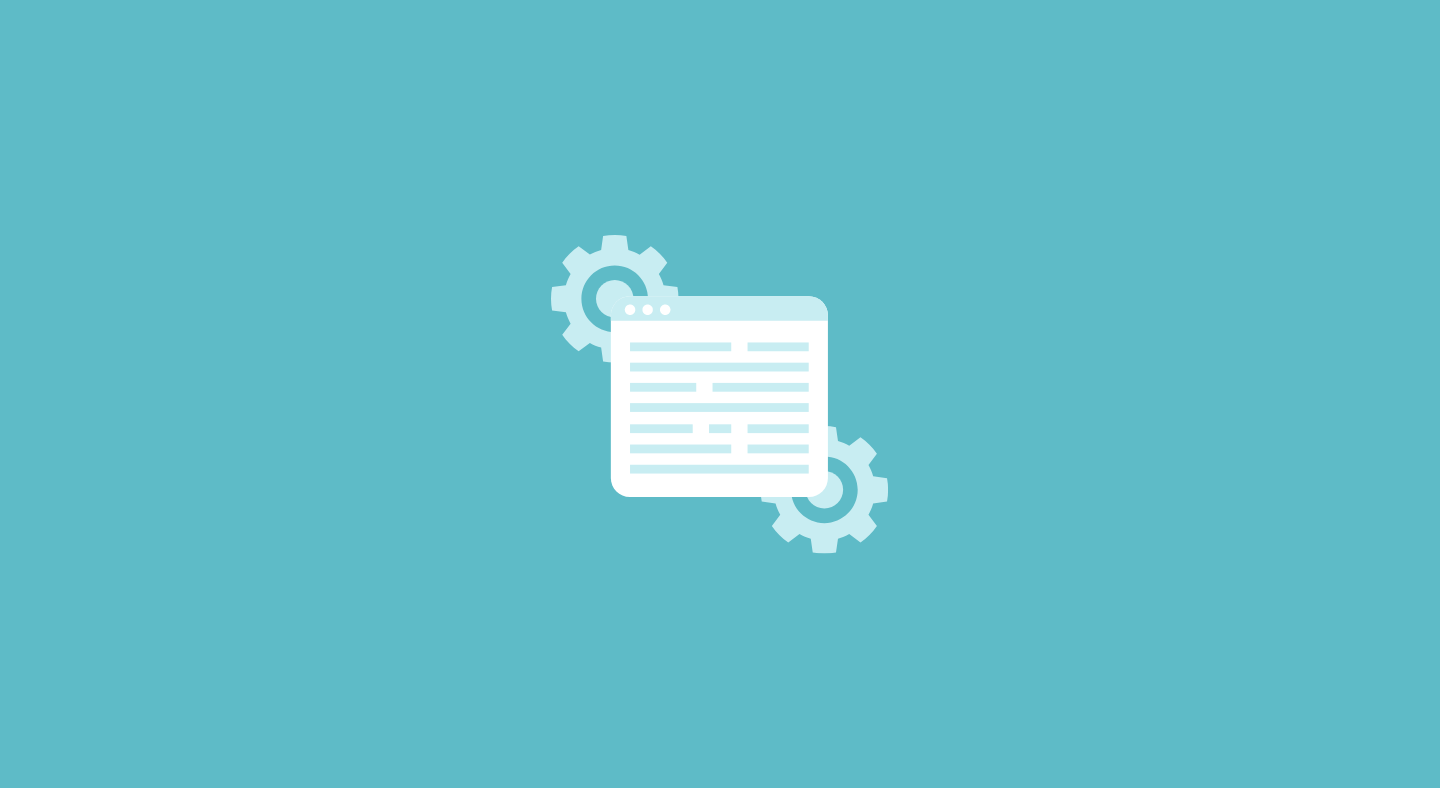
Before we begin, let me share a bit about our journey.
At Amadeus for Developers, we offer travel data and services to developers across the world through REST APIs. Thanks to HackerEarth’s platform, we recently hosted the Hack the Journey/Coding India Edition DevOps coding challenge and we invited all developers from India to participate. Since India is one of our main markets, our goal was to allow developers to explore our travel APIs and challenge their knowledge of travel solutions. We designed both theoretical and backend coding challenges, but in this article, we will focus only on the coding part.
The coding challenges were a combination of algorithmic problems and travel technologies. We asked developers to use data from the Amadeus for Developers REST APIs and our SDKs to solve them.
In this article, we will share with you the best practices to build successful Javascript coding challenges with APIs, based on our experience hosting such challenges for global developers.
Use these 6 best practices to design Javascript coding challenges
Let’s explore the 6 best practices that you need for designing Javascript coding challenges for developers:
#1—Define your objective
An essential first step is to define your objectives. When you have that clear in mind, you can build challenges to meet your goals. Some common objectives could be:
- Hiring: many companies use coding challenges as part of the recruitment process to identify and evaluate the technical skills of potential candidates.
- Awareness: companies build coding challenges to bring awareness to the developer community about their product.
- Testing your product: Javascript coding challenges are a great way to allow developers to play with your product. That can bring you valuable feedback and future ideas to improve your product roadmap.
#2—Choose the programming language
One of the key decisions you have to make when you build Javascript coding challenges: which programming language the participants will be required to use. There are several factors to take into consideration in order to identify the most fitting ones:
- Top languages: by choosing one of the top languages in the market such as Python, JavaScript, etc. you will attract a larger number of participants.
- Company-used languages: if your goal is hiring, you might want to evaluate the participants’ coding skills in your company’s programming languages
- Your familiarity with the language: make sure you are familiar with the language you offer the challenge. That will help you build challenges that fit the language and also better understand the submitted solutions.
For example, in our case, we designed both a Python and Node coding challenge since these are languages we use on a daily basis, but are also the top-used languages for our SDKs. Developers were able to choose to participate in one of these.
Also read: Top 10 Programming Languages of the Future
#3—Define the challenge
The challenge definition is one of the most crucial steps when it comes to the event’s success. Below are some points to consider:
- Difficulty level: By providing developers with some warm-up tasks and later on more challenging ones, you give them the opportunity to understand your APIs gradually. Make sure you find the right balance. To ensure the Javascript coding challenges are at the level you are thinking of, it could be useful to ask a colleague to solve them. This will give you an idea of how much time and effort is required to complete the tasks, and allow you to adjust the difficulty accordingly.
- Static data: Define challenges that the APIs always return the same data. This will help you to evaluate the solution’s correctness with the unit tests. Since that’s not easy to guarantee, you can build some sandbox environments with static data and coordinate with your API development teams to ensure that the data is not refreshed during the event.
#4—Maximize the efficiency of SDKs
If you provide SDKs as part of your API, you want developers to focus on the challenge solution and use your SDK efficiently, so we would suggest the followings:
- Set up the environment: pre-install the SDKs so developers won’t spend time and effort preparing the environment.
- Make docs accessible: to make it easy for developers to make their first API call, consider giving them the necessary resources during the challenge.
#5—Ensure API stability
Ensuring the stability of your APIs is essential to let developers solve the given challenges. Here are some points to consider:
- Check API stability: If there are any known to you instabilities (eg. backend refresh on specific days) try to avoid the event on these days. If this is not possible, inform the participants about these times to avoid potential disruptions.
- Rate limits: don’t forget to consider the API rate limits when you design the Javascript coding challenges. Provide the participants with the necessary documentation or even some helper functions to help them focus on the challenge solution.
Even if instability affects some solutions, it’s not the end of the world. If the developers have hardcoded their solution, make sure you verify their algorithm and any comments they might have left to prove that they were going to arrive at the correct answers despite the instability.
#6—Conduct unit tests
The unit tests are critical to evaluate the submissions and help you find the winners. Some best practices for the unit tests are:
- Validate API usage: in order to validate that developers indeed used your API to solve the challenge, write some unit tests to identify the usage of an API key. Also in the file that developers are going to write the code, you can pre-define some variables that are expected to add the API key and secret.
- Hide unit test files: this will ensure that developers won’t be able to know what are the expected solutions. Just a tip, it is possible for participants to get through the logs of the unit tests, and in that case, make sure you encrypt them.
Comprehensive list of JavaScript coding challenges
Delving deep into JavaScript requires a mix of theoretical knowledge and hands-on coding practice. Below are various coding challenges, organized by difficulty level and specific concepts, designed to test and improve your JavaScript prowess.
1. Beginner challenges
- Basic arithmetic operations:
- Challenge: Create a JavaScript function that takes two numbers as arguments and returns their sum, difference, product, and quotient
- Concept: Basic functions and arithmetic operationsString reversal:
- String reversal:
- Challenge: Write a JavaScript function that reverses a string
- Concept: String manipulation
- Array duplication:
- Challenge: Create a function that removes duplicates from an array
- Concept: Array manipulation and iteration
2. Intermediate challenges
- Palindrome check:
- Challenge: Determine if a given string is a palindrome (reads the same backward as forward, ignoring spaces, punctuation, and capitalization).
- Concept: String manipulation and conditional logic
- Fibonacci series:
- Challenge: Write a function that generates the first ‘n’ numbers in the Fibonacci series
- Concept: Recursion and iterative solutions
- Find the missing number:
- Challenge: Given an array containing n distinct numbers taken from 0, 1, 2, …, n, find the one that is missing from the array
- Concept: Mathematical operations and array manipulation
3. Advanced challenges
- Flatten nested array:
- Challenge: Implement a function that flattens a nested array
- Concept: Recursion and array manipulation
- Implement bind():
- Challenge: Replicate the functionality of the bind() function without using the built-in function
- Concept: Advanced functions and the ‘this’ keyword
- Deep equality check:
- Challenge: Write a function that checks if two objects (and their nested objects) are deeply equal
- Concept: Recursion, object manipulation, and deep comparison
4. Concept-specific challenges
- Promises:
- Challenge: Create a mock API call using JavaScript’s Promise
- Concept: Asynchronous programming and Promises
- Closures:
- Challenge: Design a function that generates a series of functions to add n to their argument, where n is the order in which they were generated
- Concept: Closures and function factories
- DOM Manipulation:
- Challenge: Build a simple JavaScript-based to-do list with add, delete, and mark as completed functionalities
- Concept: DOM manipulation and event handling
Additional tips for solving JavaScript coding challenges
While the right logic and approach are essential for solving coding challenges, there are several other aspects that can enhance your problem-solving journey, especially when using JavaScript. Here are some additional pointers:
- Before jumping into the code, make sure you understand the problem thoroughly. It might help to write down or discuss the problem with someone else or even talk aloud to yourself. Often, solutions emerge from a deeper understanding.
- If a problem seems too complex, break it down into smaller components or steps. This modular approach can make the overall challenge more manageable and can aid in systematic problem solving.
- JavaScript has a plethora of built-in methods, especially for arrays and strings. Familiarize yourself with these, but also know when they might be overkill. Sometimes a simpler approach might be more efficient and more readable.
- When solving a challenge, think about potential edge cases. For example, consider empty strings, arrays, or the minimum and maximum possible inputs.
- Use console.log() extensively to understand the flow of your code and to pinpoint issues. Developer tools in browsers can also provide insights into the execution of your JavaScript code.
- Your first solution doesn’t always have to be the most efficient. It’s okay to arrive at a working solution first and then iterate on it to make it better.
- Like any skill, coding gets better with regular practice. Regularly engage with coding platforms, participate in coding challenges, and always strive to learn from your mistakes.
- JavaScript, like all languages, evolves. Stay updated with the latest ECMAScript specifications and new methods or features that might be introduced.
- Join coding forums or communities where you can post your solutions and receive feedback. Sometimes, there are multiple ways to solve a problem, and seeing others’ solutions can provide new perspectives.
- Frustration can be a natural part of the problem-solving process. If you’re stuck, take a break. Sometimes, stepping away and coming back with a fresh mind can make all the difference.
Also read: How to Create a Great Take-Home Coding Test?
Create Javascript coding challenges with HackerEarth
To sum up, it’s crucial to carefully consider several factors when creating challenges that require developers to use APIs to reach the problem solution. By following best practices such as ensuring API stability, building the right unit tests, and providing necessary resources, you can create successful Javascript coding challenges that allow developers to explore and test their knowledge of your APIs. Lastly, don’t hesitate to ask HackerEarth for support and advice. Thanks to them, we were able to solve many of our doubts and build a successful coding challenge together.
We hope that these tips will be useful for your own journey.

Get advanced recruiting insights delivered every month
Related reads
The complete guide to hiring a Full-Stack Developer using HackerEarth Assessments
Fullstack development roles became prominent around the early to mid-2010s. This emergence was largely driven by several factors, including the rapid evolution of…
Best Interview Questions For Assessing Tech Culture Fit in 2024
Finding the right talent goes beyond technical skills and experience. Culture fit plays a crucial role in building successful teams and fostering long-term…
Best Hiring Platforms in 2024: Guide for All Recruiters
Looking to onboard a recruiting platform for your hiring needs/ This in-depth guide will teach you how to compare and evaluate hiring platforms…
Best Assessment Software in 2024 for Tech Recruiting
Assessment software has come a long way from its humble beginnings. In education, these tools are breaking down geographical barriers, enabling remote testing…
Top Video Interview Softwares for Tech and Non-Tech Recruiting in 2024: A Comprehensive Review
With a globalized workforce and the rise of remote work models, video interviews enable efficient and flexible candidate screening and evaluation. Video interviews…
8 Top Tech Skills to Hire For in 2024
Hiring is hard — no doubt. Identifying the top technical skills that you should hire for is even harder. But we’ve got your…
